Hiển thị sản phẩm trong danh mục sản phẩm khi click
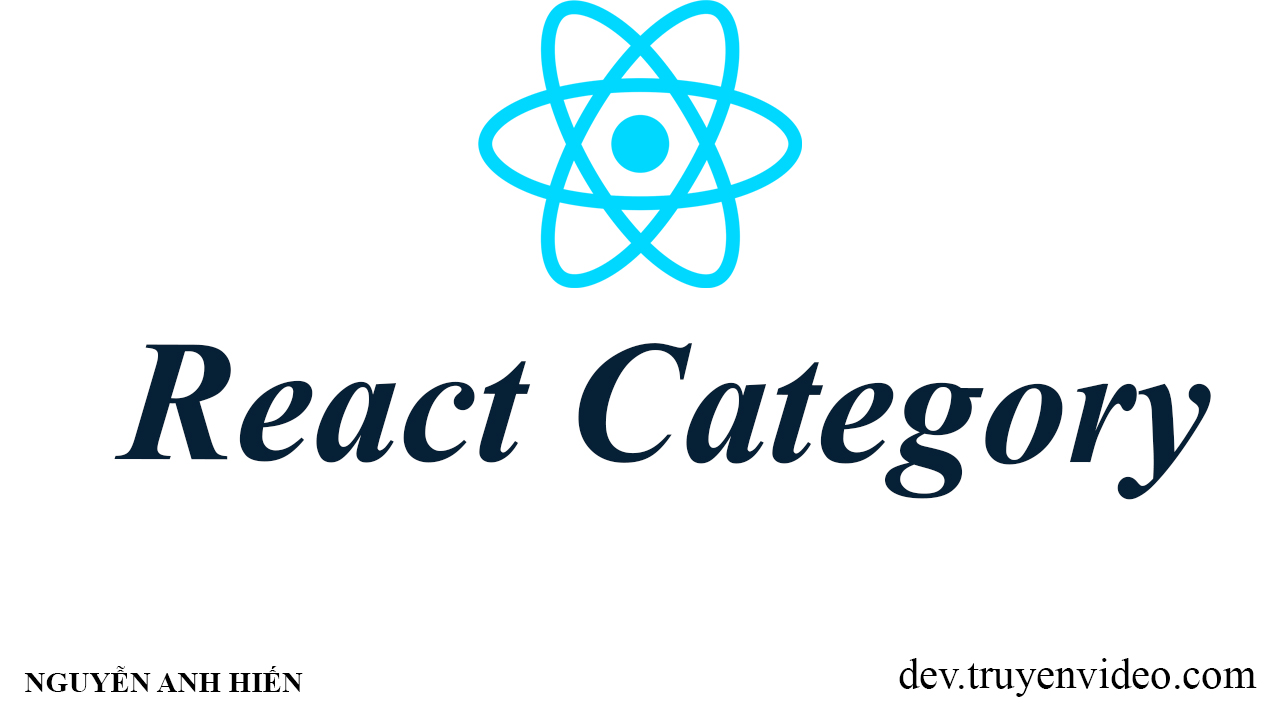
Đầu tiên để hiển thị sản phẩm trong danh mục sản phẩm khi lập trình react chúng ta cần tạo 1 project với command sau :
npm init vite@latest
Chọn ngôn ngữ react rồi next và cài đặt như bình thường
File App.tsx
import { useState } from "react";
import reactLogo from "./assets/react.svg";
import viteLogo from "/vite.svg";
import "./App.css";
import ListSanpham from "./component/ListSanpham";
import { useRecoilValue } from "recoil";
import { totalCart } from "./state/SanphamState";
import Danhmucsanpham from "./component/Danhmucsanpham";
function App() {
const total_gia = useRecoilValue(totalCart);
return (
<>
<Danhmucsanpham />
<ListSanpham />
<h3>{total_gia}</h3>
</>
)
}
export default App;
Trong file \src\component\Danhmucsanpham.tsx
import React from "react";
import { useRecoilState } from "recoil";
import { DanhmucState } from "../state/DanhmucState";
const Danhmucsanpham = () => {
let list_danhmuc = [
{ name: "Danh mục 1", id: 1, des: 'Đây là miêu tả 1' },
{ name: "Danh mục 2", id: 2, des: 'Đây là miêu tả 2' },
];
const [danhmuc, setDanhmuc] = useRecoilState(DanhmucState);
const SelectCate = (id = 0) => {
setDanhmuc(id);
}
return (
<div>
{list_danhmuc.map(item => (
<div key={item.id}>
<button onClick={() => SelectCate(item.id)}>
<h3>{item.name}</h3>
</button>
</div>
))}
</div>
)
}
export default Danhmucsanpham;
Trong file src\component\ListSanpham.tsx
import React, { useEffect, useState } from "react";
import { Container, Col } from "react-bootstrap";
import { useRecoilState } from "recoil";
import { addtoCart, movetoCart } from "../func/Giohang";
import { DanhmucState } from "../state/DanhmucState";
import { CartState, SanphamState } from "../state/SanphamState";
function ListSanpham() {
let list_products = [
{ name: "sản phẩm 1", id: 1, des: 'Đây là miêu tả 1', cost: 10000, category: 1 },
{ name: "sản phẩm 2", id: 2, des: 'Đây là miêu tả 2', cost: 14000, category: 1 },
{ name: "sản phẩm 3", id: 3, des: 'Đây là miêu tả 3', cost: 12000, category: 2 },
{ name: "sản phẩm 4", id: 4, des: 'Đây là miêu tả 4', cost: 12000, category: 2 },
];
const [danhmuc, setDanhmuc] = useRecoilState(DanhmucState);
const [products, setProducts] = useRecoilState(SanphamState);
const [cart, setCart] = useRecoilState(CartState);
useEffect(() => {
if (danhmuc === 0) {
if (products.length < 1) {
setProducts(list_products);
}
} else {
let new_list_products = [];
list_products.forEach(product => {
if (product.category === danhmuc) {
new_list_products.push(product);
}
})
setProducts(new_list_products);
//console.log(sessionStorage.getItem('products'));
}
}, [danhmuc])
return (
<>
<Container fluid>
<div>
{products.map(product => (
<div key={product.id}>
<button onClick={() => setCart(addtoCart(cart, product))}>Thêm vào giỏ hàng</button>
<p>{product.name}</p>
<button onClick={() => setCart(movetoCart(cart, product))}>Xóa khỏi giỏ hàng</button>
</div>
))}
</div>
</Container>
</>
)
}
export default ListSanpham
Các hàm function liên quan đến xử lý thêm và xóa sản phẩm \src\func\Giohang.tsx
import { useRecoilState, useSetRecoilState } from "recoil";
import { CartState } from "../state/SanphamState";
export const addtoCart = (cart: any, sanpham: any) => {
let newCart = [...cart];
let vitri = -1;
for (let i = 0; i < newCart.length; i++) {
if (newCart[i].sanpham.id === sanpham.id) {
vitri = i;
break;
}
};
if (vitri === -1) {
let item = { id: sanpham.id, sanpham: sanpham, soluong: 1, cost: sanpham.cost };
newCart.push(item);
} else {
newCart[vitri] = {
// ...cart[vitri],
id: cart[vitri].id,
sanpham: cart[vitri].sanpham,
cost: cart[vitri].cost,
soluong: cart[vitri].soluong + 1,
};
}
return newCart;
}
export const movetoCart = (cart: any, sanpham: any) => {
let newCart = [...cart];
let vitri = -1;
for (let i = 0; i < newCart.length; i++) {
if (newCart[i].sanpham.id === sanpham.id) {
vitri = i;
break;
}
};
if (vitri === -1) {
alert('Không có sản phẩm này trong giỏ hàng');
return cart;
} else {
if (cart[vitri].soluong > 1) {
newCart[vitri] = {
// ...cart[vitri],
id: cart[vitri].id,
sanpham: cart[vitri].sanpham,
cost: cart[vitri].cost,
soluong: cart[vitri].soluong - 1,
};
} else {
newCart.splice(vitri, 1);
}
return newCart;
}
}
State ta code như sau :
import React from "react";
import { atom, selector } from 'recoil';
export const SanphamState = atom({
key: 'SanphamState',
default: [],
});
export const CartState = atom({
key: 'CartState',
default: [],
});
export const totalCart = selector({
key: 'totalCart',
get: ({ get }) => {
const cart = get(CartState);
let total = 0;
if (cart) {
cart.forEach((item) => {
total += item.cost * item.soluong;
});
}
return total;
},
})
export const DanhmucState = atom({
key: 'DanhmucState',
default: 0,
});
Link demo mình để cuối bài